The AskHandle Blog
Explore articles on the latest advancements in AI innovation, customer experience and modern lifestyle!
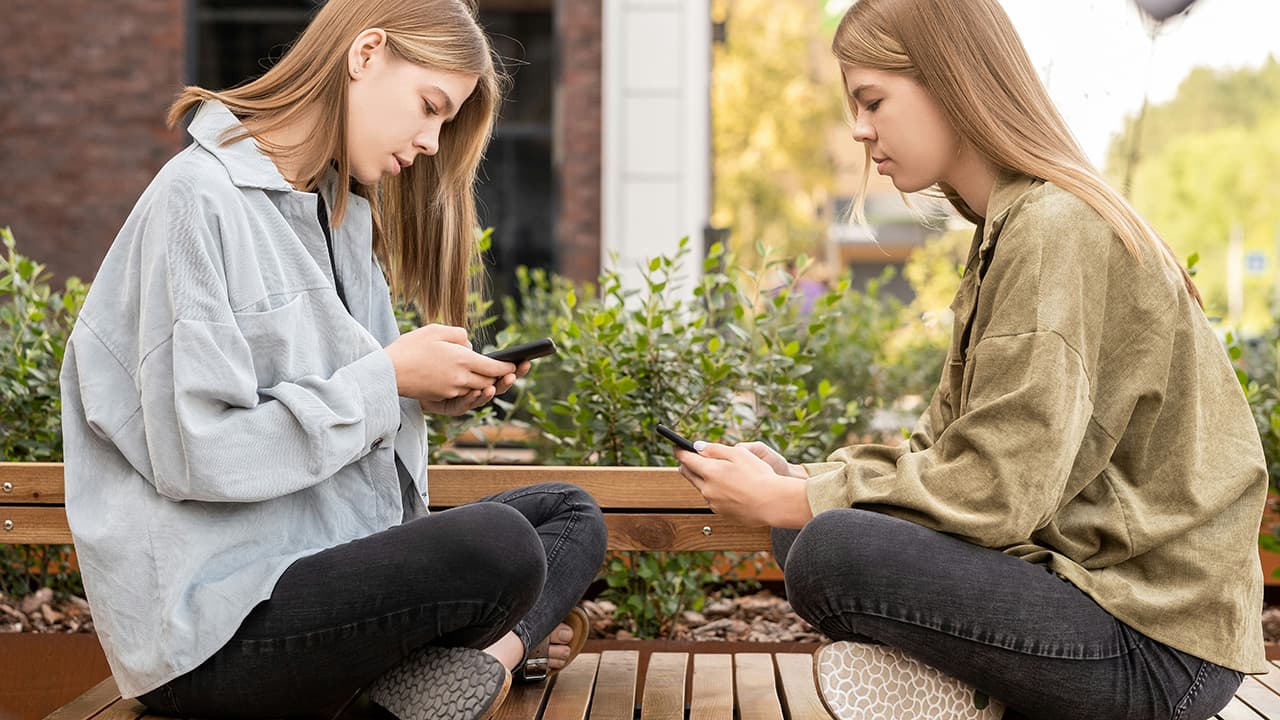
Top Personal Communication Channels in the U.S. (2025 Edition)
In today’s hyper-connected world, how we message each other says a lot about our culture, devices, and even our age group. While social apps come and go, certain platforms dominate personal communication in the United States. If you're curious about what Americans are using to stay in touch — especially for person-to-person messaging — here’s a breakdown of the top personal communication channels in 2025.
Written byNicole Davids
Published onMay 9, 2025
- View all